https://qiita.com/BEIKE/items/825e62bbd8d92b6d347e
git clone https://github.com/shilohc/map2gazebo.git cd ~/catkin_ws && catkin build source ~/catkin_ws/devel/setup.bash
roslaunch map2gazebo map2gazebo.launch params_file:=$(rospack find map2gazebo)/config/defaults.yaml
rosrun map_server map_server map.yaml
zotac-d05@d05:~/catkin_ws/src/halc_simulation/map$ rosrun map_server map_server halc0311_a.yaml
[ERROR] [1741507226.788502]: bad callback: <bound method MapConverter.map_callback of <__main__.MapConverter object at 0x7fd8238e8f40>> Traceback (most recent call last): File "/opt/ros/noetic/lib/python3/dist-packages/rospy/topics.py", line 750, in _invoke_callback cb(msg) File "/home/zotac-d05/catkin_ws/src/map2gazebo/src/map2gazebo.py", line 30, in map_callback contours = self.get_occupied_regions(map_array) File "/home/zotac-d05/catkin_ws/src/map2gazebo/src/map2gazebo.py", line 72, in get_occupied_regions image, contours, hierarchy = cv2.findContours( ValueError: not enough values to unpack (expected 3, got 2)
- First we need to install these libraries
pip install –user trimesh
pip install –user numpy
pip install –user pycollada
pip install –user scipy
pip install –user networkx
2. Then change open function variable from “w” to “wb”
if mesh_type == "stl":
with open(export_dir + "/map.stl", 'wb') as f:
mesh.export(f, "stl")
rospy.loginfo("Exported STL. You can shut down this node now")
elif mesh_type == "dae":
with open(export_dir + "/map.dae", 'wb') as f:
f.write(trimesh.exchange.dae.export_collada(mesh))
3. You can select output between stl or dae
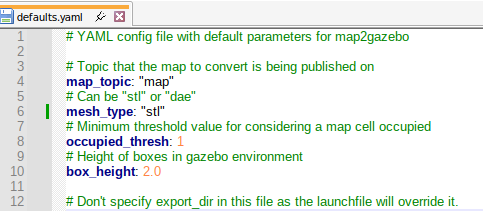
4. The export directory was set to $(find map2gazebo)/models/map/meshes” if there is no this folder, it will say the error…
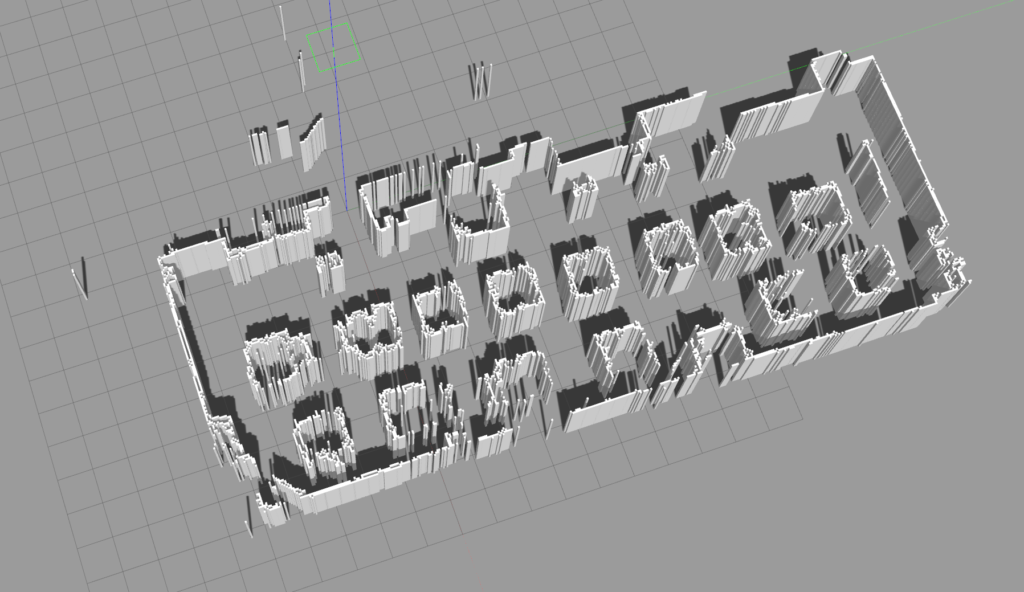
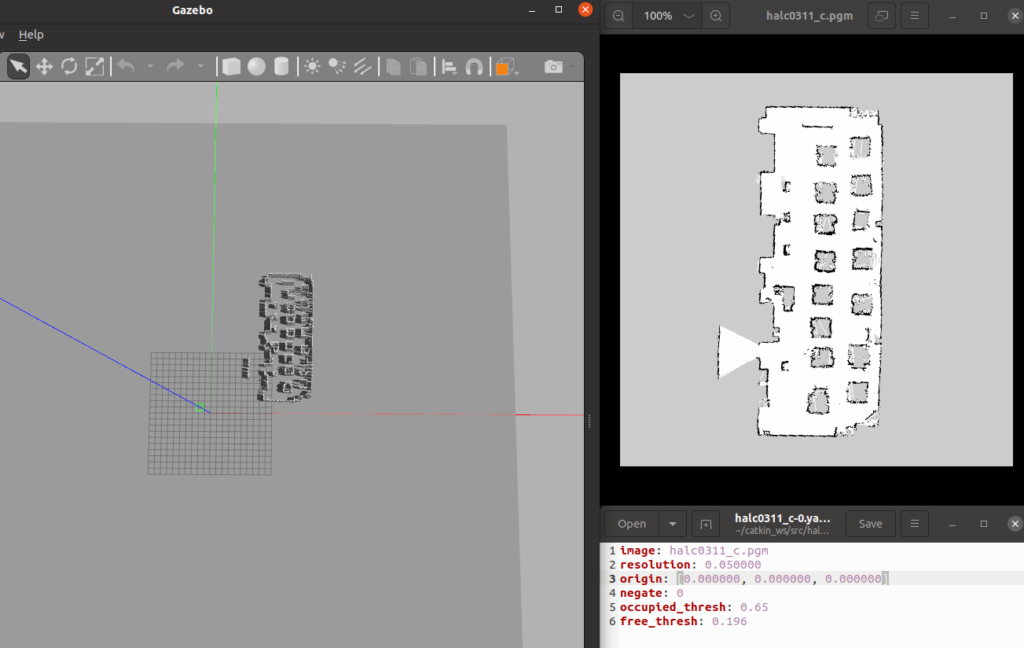
that reflect gazebo world
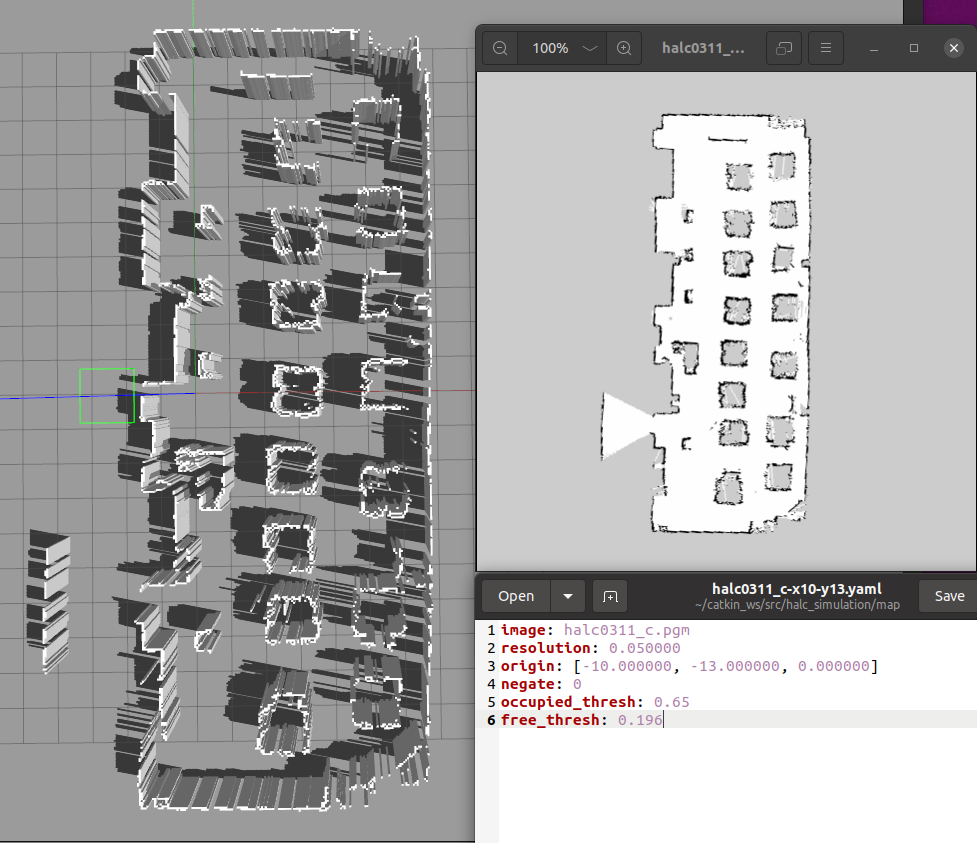
Then, File -> Save World As halc.world
hence you will get the current camera pose.
copy the models folder to the package where you copy .world to
<launch>
<env name="GAZEBO_MODEL_PATH" value="$(find map2gazebo)/models:$(optenv GAZEBO_MODEL_PATH)" />
<arg name="debug" default="false"/>
<arg name="gui" default="true"/>
<arg name="headless" default="false"/>
<!-- Start Gazebo with a blank world -->
<include file="$(find gazebo_ros)/launch/empty_world.launch">
<arg name="debug" value="$(arg debug)" />
<arg name="gui" value="$(arg gui)" />
<arg name="paused" value="false"/>
<arg name="use_sim_time" value="true"/>
<arg name="headless" value="$(arg headless)"/>
<arg name="world_name" value="$(find map2gazebo)/worlds/map.sdf"/>
</include>
</launch>
<?xml version="1.0" ?>
<sdf version="1.4">
<model name="map">
<link name="link">
<inertial>
<mass>15</mass>
<inertia>
<ixx>0.0</ixx>
<ixy>0.0</ixy>
<ixz>0.0</ixz>
<iyy>0.0</iyy>
<iyz>0.0</iyz>
<izz>0.0</izz>
</inertia>
</inertial>
<collision name="collision">
<pose>0 0 0 0 0 0</pose>
<geometry>
<mesh>
<uri>model://map/meshes/map.stl</uri>
</mesh>
</geometry>
</collision>
<visual name="visual">
<pose>0 0 0 0 0 0</pose>
<geometry>
<mesh>
<uri>model://map/meshes/map.stl</uri>
</mesh>
</geometry>
</visual>
</link>
<static>1</static>
</model>
</sdf>
zotac-d05@d05:~/catkin_ws/src$ catkin_create_pkg halc_simulation Created file halc_simulation/package.xml Created file halc_simulation/CMakeLists.txt Successfully created files in /home/zotac-d05/catkin_ws/src/halc_simulation. Please adjust the values in package.xml. zotac-d05@d05:~/catkin_ws/src$ cd halc_simulation zotac-d05@d05:~/catkin_ws/src/halc_simulation$ mkdir -p worlds launch
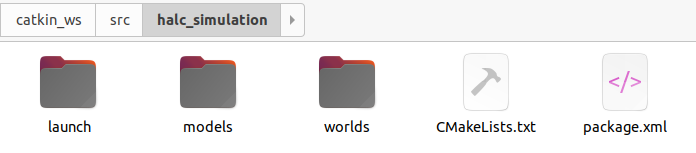
zotac-d05@d05:~/catkin_ws$ catkin build
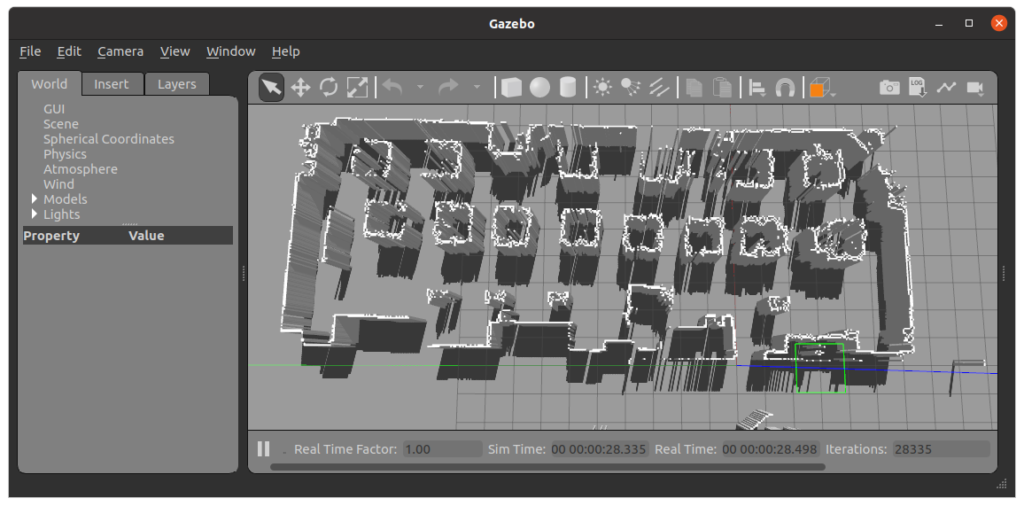
Creating a world with turtlebot (this method load map.sdf, unable to adjust the camera pose, just save the world via gazebo is fine!)
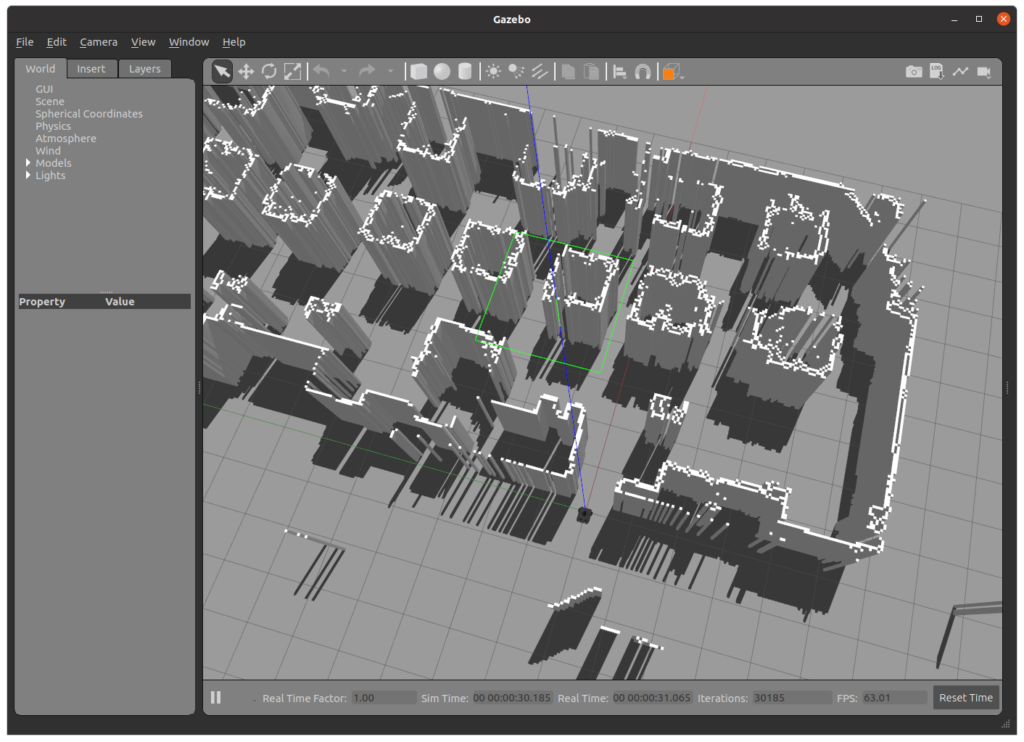
<launch>
<arg name="model" default="$(env TURTLEBOT3_MODEL)" doc="model type [burger, waffle, waffle_pi]"/>
<arg name="x_pos" default="0.0"/>
<arg name="y_pos" default="0.0"/>
<arg name="z_pos" default="0.0"/>
<arg name="yaw" default="0.0"/>
<env name="GAZEBO_MODEL_PATH" value="$(find halc_simulation)/models:$(optenv GAZEBO_MODEL_PATH)" />
<!-- Start Gazebo with a blank world -->
<include file="$(find gazebo_ros)/launch/empty_world.launch">
<arg name="world_name" value="$(find halc_simulation)/worlds/map.sdf"/>
<arg name="paused" value="false"/>
<arg name="use_sim_time" value="true"/>
<arg name="gui" value="true"/>
<arg name="headless" value="false"/>
<arg name="debug" value="false"/>
</include>
<param name="robot_description" command="$(find xacro)/xacro --inorder $(find turtlebot3_description)/urdf/turtlebot3_$(arg model).urdf.xacro" />
<node pkg="gazebo_ros" type="spawn_model" name="spawn_urdf" args="-urdf -model turtlebot3_$(arg model) -x $(arg x_pos) -y $(arg y_pos) -z $(arg z_pos) -param robot_description" />
</launch>
Use this kind of launch file is better!
<launch>
<arg name="model" default="$(env TURTLEBOT3_MODEL)" doc="model type [burger, waffle, waffle_pi]"/>
<arg name="x_pos" default="0.0"/>
<arg name="y_pos" default="0.0"/>
<arg name="z_pos" default="0.0"/>
<arg name="yaw" default="0.0"/>
<env name="GAZEBO_MODEL_PATH" value="$(find halc_simulation)/models:$(optenv GAZEBO_MODEL_PATH)" />
<!-- Start Gazebo with a blank world -->
<include file="$(find gazebo_ros)/launch/empty_world.launch">
<arg name="world_name" value="$(find halc_simulation)/worlds/halc.world"/>
<arg name="paused" value="false"/>
<arg name="use_sim_time" value="true"/>
<arg name="gui" value="true"/>
<arg name="headless" value="false"/>
<arg name="debug" value="false"/>
</include>
<param name="robot_description" command="$(find xacro)/xacro --inorder $(find turtlebot3_description)/urdf/turtlebot3_$(arg model).urdf.xacro" />
<node pkg="gazebo_ros" type="spawn_model" name="spawn_urdf" args="-urdf -model turtlebot3_$(arg model) -x $(arg x_pos) -y $(arg y_pos) -z $(arg z_pos) -param robot_description" />
</launch>
roslaunch turtlebot3_teleop turtlebot3_teleop_key.launch
roslaunch halc_simulation turtlebot3_gazebo_halc.launch
roslaunch turtlebot3_slam turtlebot3_slam.launch slam_methods:=gmapping
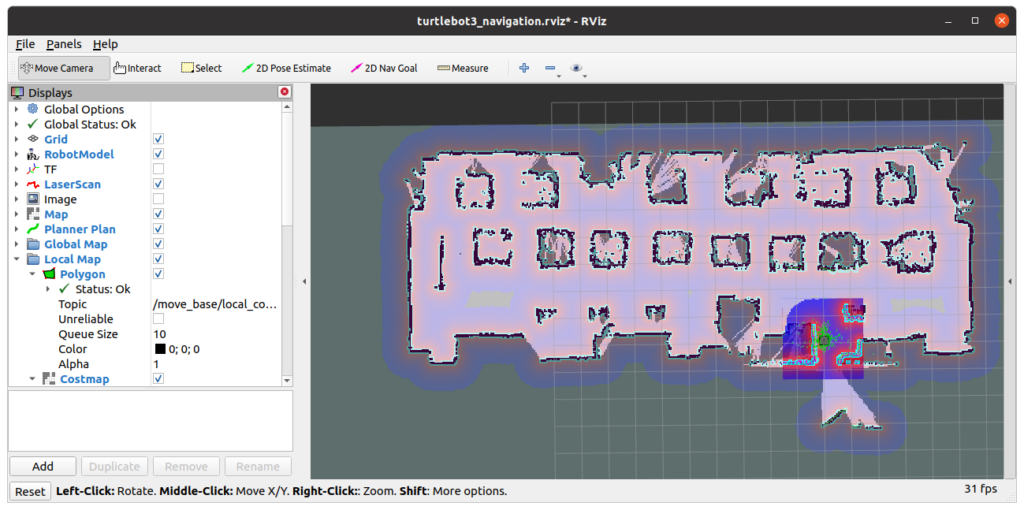
zotac-d05@d05:~$ rostopic echo /amcl_pose | sed -n -e '/pose:/,+10p' pose: pose: position: x: 2.6776955645384244 y: 0.006516339994905264 z: 0.0 orientation: x: 0.0 y: 0.0 z: 0.04651613535574757 w: 0.9989175387145657
roslaunch halc_simulation turtlebot3_gazebo_halc.launch
roslaunch turtlebot3_navigation turtlebot3_navigation.launch map_file:=$HOME/catkin_ws/src/halc_simulation/map/halc_map.yaml
rostopic echo /move_base/status | sed -n -e ‘/seq:/p’ -e ‘/status:/p’ -e ‘/text:/p’
zotac-d05@d05:~/catkin_ws/src/halc_simulation$
rosrun halc_simulation megarover_sequence_goals.py goal_halc_3.yaml