Import HoloToolkit 2017.4.3.0
Menu : Mixed Reality Toolkit > Configure > Apply Mixed Reality Project Setting > Check “Use Toolkit-specific InputManager axes”
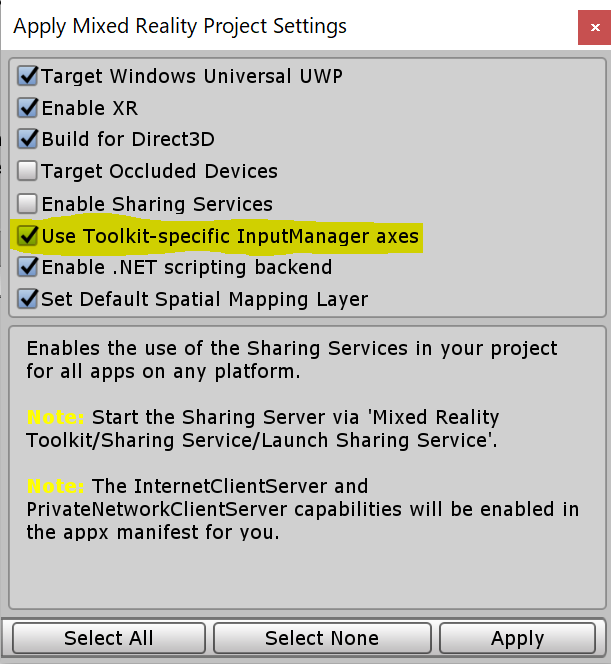
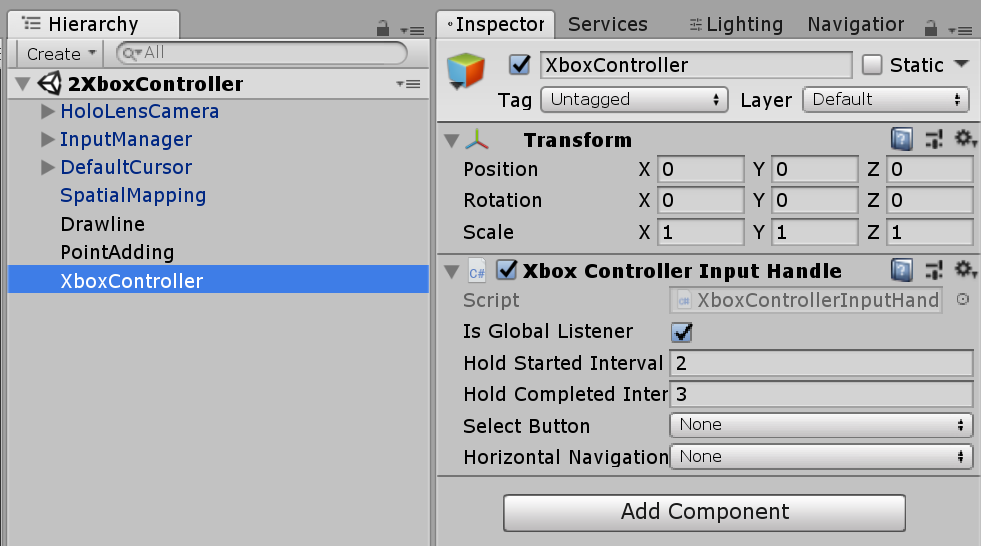
using System;
using HoloToolkit.Unity.InputModule;
public class XboxControllerInputHandle : XboxControllerHandlerBase
{
public event Action On_Y_ButtonPressed = delegate { };
public event Action On_B_ButtonPressed = delegate { };
public event Action On_X_ButtonPressed = delegate { };
public override void OnXboxInputUpdate(XboxControllerEventData eventData)
{
base.OnXboxInputUpdate(eventData);
//XboxA_Down overlap with the default select
if (eventData.XboxY_Down)
{
On_Y_ButtonPressed();
}
if (eventData.XboxB_Down)
{
On_B_ButtonPressed();
}
if (eventData.XboxX_Down)
{
On_X_ButtonPressed();
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DrawLineByPoints : MonoBehaviour
{
private LineRenderer lr;
private List<Vector3> points;
public bool visible { get; private set; }
private void Awake()
{
visible = true;
lr = GetComponent<LineRenderer>();
points = new List<Vector3>();
}
public void Hide()
{
visible = false;
gameObject.SetActive(false);
}
public void Show()
{
visible = true;
gameObject.SetActive(true);
}
public void SetUpLine(List<Vector3> points)
{
lr.positionCount = points.Count;
this.points = points;
}
private void Update()
{
for(int i=0;i<points.Count;i++)
{
lr.SetPosition(i,points[i]);
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using HoloToolkit.Unity.InputModule;
public class PointAdding : MonoBehaviour
{
private List<Vector3> positions;
[SerializeField] private DrawLineByPoints line;
[SerializeField] private XboxControllerInputHandle XBoxInputHandleScript;
void Start()
{
positions = new List<Vector3>();
InputManager.Instance.PushFallbackInputHandler(gameObject);
XBoxInputHandleScript.On_Y_ButtonPressed += Handle_Y_ButtonPressed;
XBoxInputHandleScript.On_B_ButtonPressed += Handle_B_ButtonPressed;
XBoxInputHandleScript.On_X_ButtonPressed += Handle_X_ButtonPressed;
}
private void Handle_Y_ButtonPressed()
{
if(line.visible) line.Hide();
else line.Show();
}
private void Handle_B_ButtonPressed()
{
Vector3 hitPoint = GazeManager.Instance.HitPosition;
positions.Add(hitPoint);
Debug.Log("Click on " + hitPoint.ToString());
line.SetUpLine(positions);
}
private void Handle_X_ButtonPressed()
{
positions.Clear();
line.SetUpLine(positions);
}
}
Press B : add new point for drawing a line
Press X : remove all points
Press Y : Show/Hide the line
Note that the A button is a select action by default.