Each airtap do: create object, add rigidbody (gravity) to the object, and finally delete the object.
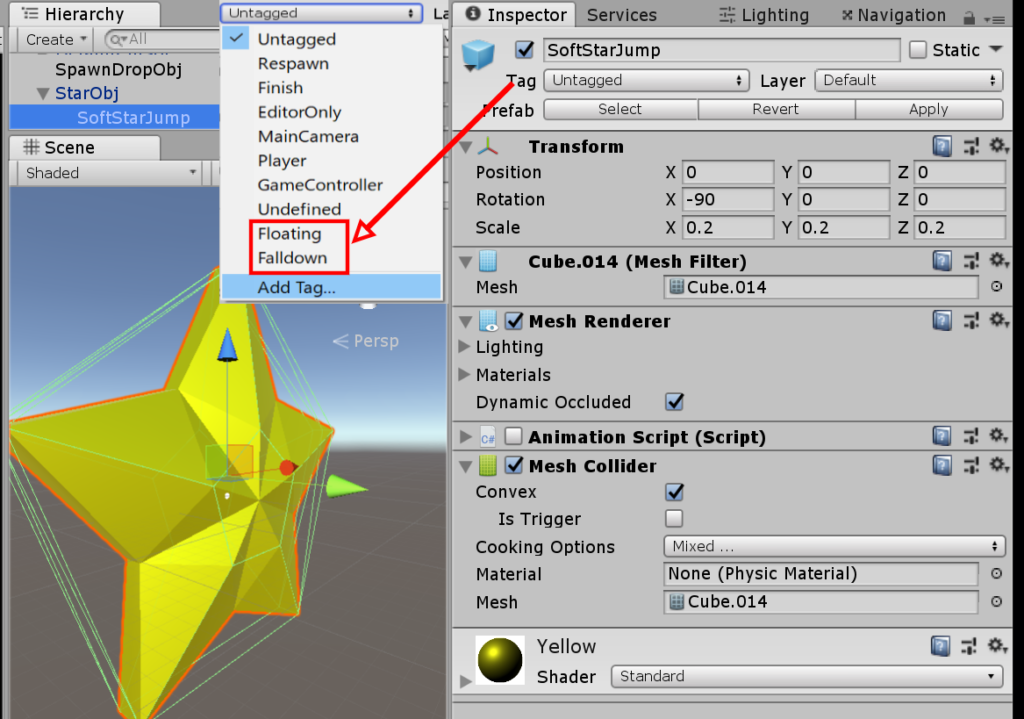
Somehow, the GazeManager.Instance.HitObject hit the child obj that contain a mesh/box collider. Therefore, we need to check tag name on its parent.
Note that, Mesh Collider needs to check on Convex, otherwise when add a rigidbody, it will fall down eternity without colliding with the room floor.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using HoloToolkit.Unity.InputModule;
public class SpawnDropObject : MonoBehaviour, IInputClickHandler
{
//[SerializeField] private PanelDebug panelDebug;
public GameObject iprefab;
private int ObjCount;
private List<GameObject> ObjList;
void Start()
{
InputManager.Instance.PushFallbackInputHandler(gameObject);
ObjList = new List<GameObject>();
}
public void OnInputClicked(InputClickedEventData eventData)
{
if (!GazeManager.Instance.HitObject) //airtap at nothing = create new obj
{
Debug.Log("!GazeManager.Instance.HitObject");
Vector3 obj_position = Camera.main.transform.position + Camera.main.transform.forward;
CreateNewObject(obj_position);
}
else
{
//panelDebug.ShowMessage(GazeManager.Instance.HitObject.name);
Debug.Log("\n"+GazeManager.Instance.HitObject.name + " " + GazeManager.Instance.HitObject.tag + " " + GazeManager.Instance.HitObject.transform.parent.tag);
// Airtap at floating object. Then, add gravity to the obj = drop it down
if (GazeManager.Instance.HitObject.tag == "Floating")
{
Debug.Log("HitObject.tag == Floating");
GazeManager.Instance.HitObject.AddComponent<Rigidbody>();
GazeManager.Instance.HitObject.tag = "Falldown";
}
else if (GazeManager.Instance.HitObject.transform.parent.tag == "Floating")
{
Debug.Log("HitObject.parent.tag == Floating");
GazeManager.Instance.HitObject.AddComponent<Rigidbody>();
GazeManager.Instance.HitObject.transform.parent.tag = "Falldown";
}
// Airtap at object on floor. Then, remove it.
else if (GazeManager.Instance.HitObject.tag == "Falldown")
{
Debug.Log("HitObject.tag == Falldown");
ObjList.Remove(GazeManager.Instance.HitObject);
Destroy(GazeManager.Instance.HitObject);
}
else if (GazeManager.Instance.HitObject.transform.parent.tag == "Falldown")
{
Debug.Log("HitObject.parent.tag == Falldown");
ObjList.Remove(GazeManager.Instance.HitObject.transform.parent.gameObject);
Destroy(GazeManager.Instance.HitObject.transform.parent.gameObject);
}
// Airtap at something (room mesh). Then, create new obj.
else
{
Debug.Log("HitObject.tag == ??");
Debug.Log("HitObject" + GazeManager.Instance.HitObject.transform.position.ToString());
Debug.Log("HitPosition" + GazeManager.Instance.HitObject.ToString());
//CreateNewObject(GazeManager.Instance.HitObject.transform.position); // this position is not the world coordinate
CreateNewObject(GazeManager.Instance.HitPosition);
}
}
string objlistname = "\nObj in List";
foreach (GameObject obj in ObjList)
{
string text = "\n"+obj.name + " " + obj.tag;
objlistname += text;
}
Debug.Log(objlistname);
}
private void CreateNewObject(Vector3 position)
{
Debug.Log("CreateNewObject at"+ position.ToString());
GameObject newobj = Instantiate(iprefab, position, Quaternion.identity);
newobj.tag = "Floating";
ObjList.Add(newobj);
}
}