This application will record Hololens transformation overtime and save to any text file (my code is csv file) inside the device.
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
using System;
using System.IO;
using System.Linq;
#if WINDOWS_UWP
using Windows.Storage;
using Windows.System;
using System.Threading.Tasks;
using Windows.Storage.Streams;
#endif
// saved folder : User Folders \ LocalAppData \ Appname \ LocalState \
public class WriteCSVFile : MonoBehaviour
{
#if WINDOWS_UWP
Windows.Storage.ApplicationDataContainer localSettings = Windows.Storage.ApplicationData.Current.LocalSettings;
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
#endif
private string timeStamp;
private string fileName;
private string saveInformation;
public void WriteTextToFile(string text)
{
timeStamp = System.DateTime.Now.ToString().Replace("/", "_").Replace(":", "-").Replace(" ", "_");
fileName = "transform-" + timeStamp + ".csv";
saveInformation = text;
#if WINDOWS_UWP
WriteData();
#endif
}
#if WINDOWS_UWP
async void WriteData()
{
StorageFile saveFile = await localFolder.CreateFileAsync(fileName, CreationCollisionOption.ReplaceExisting);
string fileheader = "id,pos_x,pos_y,pos_z,rot_x,rot_y,rot_z" + "\r\n";
await FileIO.AppendTextAsync(saveFile, fileheader + saveInformation);
}
#endif
}
In another script file, we record transform data and call WriteTextToFile() to write a text.
[SerializeField] private WriteCSVFile writeCSVFile;
private StringBuilder csv;
private void PreparePositiontosave()
{
csv.Remove(0, csv.Length);
for (int i = 0; i < positions.Count; i++)
{
var newLine = string.Format("{0},{1},{2},{3},{4},{5},{6}", i,
positions[i].x, positions[i].y, positions[i].z,
rotations[i].x, rotations[i].y, rotations[i].z);
csv.AppendLine(newLine);
writeCSVFile.WriteTextToFile(csv.ToString());
}
}
Here is where the file was saved and its content.
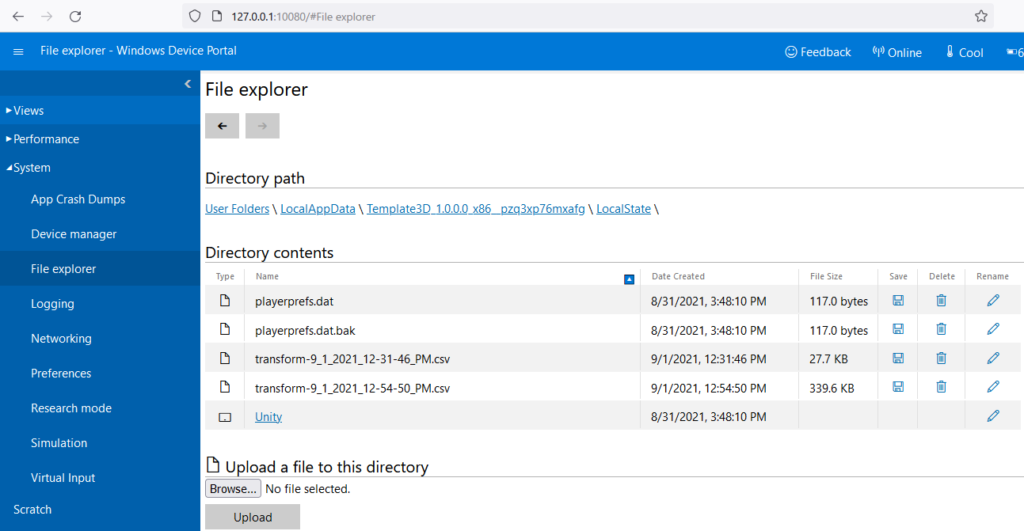
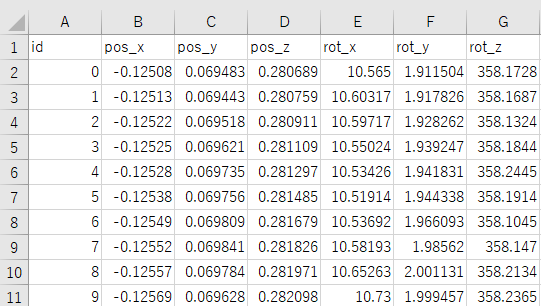